How to Use Open AI ChatGPT API in Python
Incorporating OpenAI’s ChatGPT API into Python applications can significantly enhance their conversational abilities. This article provides a step-by-step guide on connecting to the API, customizing outputs, and implementing advanced features like conversational bots and efficient API usage.
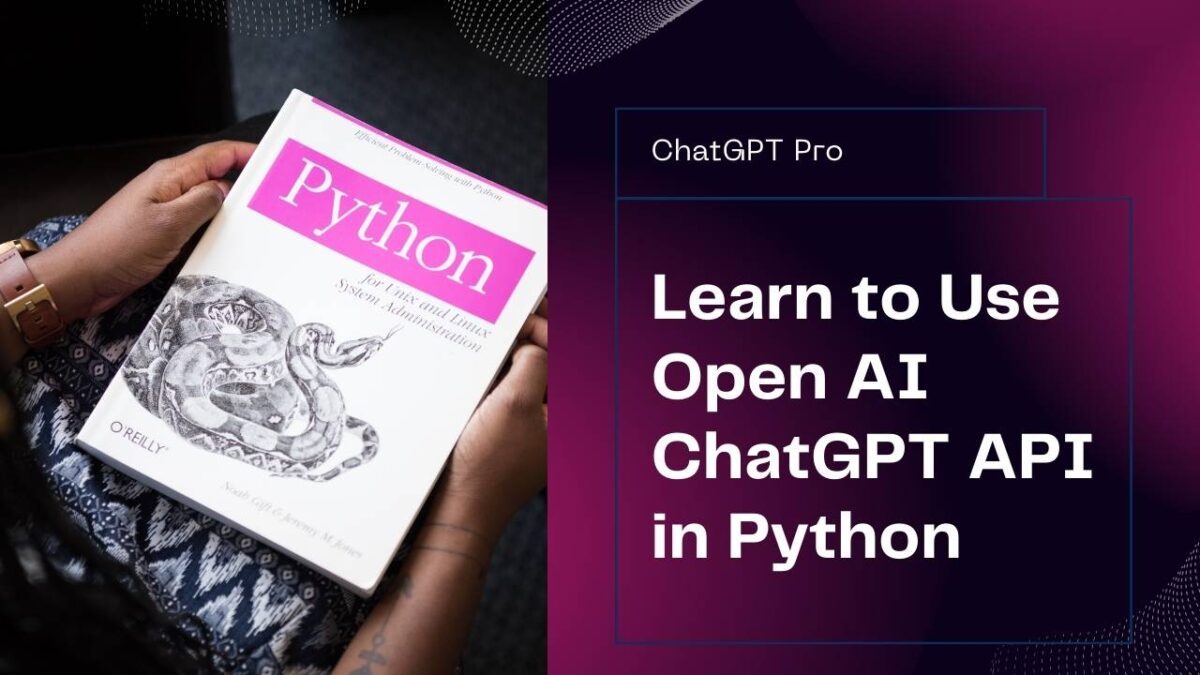
By following this tutorial, developers will be equipped to create intelligent, responsive applications that engage users effectively.
Check out: Free Twitter Thread Generator
What is Open AI ChatGPT API?
The ChatGPT API, developed by OpenAI, serves as a bridge for developers to integrate advanced conversational AI into their applications. By providing access to models like GPT-4o, it enables the generation of human-like text responses, enhancing user interactions across various platforms.
Key features of the ChatGPT API include natural language understanding, context retention, and adaptability. These capabilities allow applications to interpret and respond to user inputs in a manner that feels intuitive and engaging. The API’s flexibility supports a wide range of applications, from simple queries to complex dialogues.
The versatility of the ChatGPT API is evident in its diverse use cases. In chatbot development, it powers virtual assistants capable of addressing customer inquiries, scheduling appointments, and providing personalized recommendations.
For content generation, it assists in drafting emails, writing articles, and creating marketing materials, streamlining workflows for content creators. In data analysis, the API aids in interpreting datasets and generating comprehensive reports, making data-driven insights more accessible.
Setting Up the Environment for Open AI ChatGPT API in Python
To integrate the ChatGPT API into a Python project, one must first establish the appropriate environment. This involves ensuring Python is installed and obtaining an API key from OpenAI.
Subsection 3.1: Prerequisites
Begin by installing Python. Visit the official Python downloads page and select the version compatible with your operating system. The website provides installers for Windows, macOS, and Linux. After downloading, run the installer and follow the on-screen instructions. It’s advisable to check the option to add Python to your system’s PATH during installation, as this facilitates command-line operations.
Next, register for an API key on OpenAI’s platform. Navigate to the OpenAI API key page and log in or create an account if you haven’t already. Once logged in, click on “Create new secret key” to generate your unique API key. Ensure you store this key securely, as it grants access to OpenAI’s services.
Subsection 3.2: Installing Required Libraries
With Python installed and your API key ready, the next step is to install the necessary Python libraries. The openai
library is essential for interacting with the ChatGPT API. To install it, open your command-line interface and execute the following command:
bashCopy codepip install openai
This command utilizes pip
, Python’s package installer, to download and install the openai
library from the Python Package Index (PyPI). If pip
isn’t already installed, you can find installation instructions in the Python documentation.
After installing the openai
library, it’s prudent to verify the installation. You can do this by opening a Python interpreter and attempting to import the library:
pythonCopy codeimport openai
If no errors occur, the installation was successful, and you can proceed to develop your application using the ChatGPT API.
Understanding the Open AI ChatGPT API Structure
The ChatGPT API offers developers a suite of endpoints and parameters to tailor AI-generated responses to specific needs. Understanding these components is essential for effective integration.
API Endpoints
The primary endpoint for interacting with ChatGPT is the /v1/chat/completions
endpoint. This endpoint facilitates the generation of conversational responses based on provided prompts. Developers send a POST request to this endpoint, including necessary parameters in the request body.
Key Parameters
- Prompt: This parameter, often referred to as
messages
, is an array of dictionaries representing the conversation history. Each dictionary includes arole
(such as ‘user’, ‘system’, or ‘assistant’) andcontent
(the text of the message). This structure allows the model to understand the context and generate appropriate responses. - Max Tokens: Defined by the
max_tokens
parameter, this sets the upper limit on the number of tokens the model can generate in a response. Tokens are chunks of text; for example, the word “fantastic” might be split into “fan”, “tas”, and “tic”. Setting this limit helps control the length of the output and manage costs, as API usage is often token-based. - Temperature: This parameter influences the randomness of the model’s responses. A lower temperature (e.g., 0.2) results in more deterministic outputs, making the model’s responses more focused and predictable. Conversely, a higher temperature (e.g., 0.8) introduces more randomness, which can be useful for creative tasks where diverse outputs are desired.
- API Model Versions: OpenAI provides various model versions, such as
gpt-4o,gpt-4o-mini
andgpt-4
. Each version offers different capabilities and performance characteristics. Selecting the appropriate model version depends on the specific requirements of the application, balancing factors like response quality and computational resources.
Error Handling and Rate Limiting
When integrating the ChatGPT API, it’s important to implement robust error handling to manage potential issues such as network errors, invalid inputs, or exceeded rate limits. OpenAI enforces rate limits to ensure fair usage across all users.
These limits include restrictions on the number of requests per minute and the number of tokens processed per minute.
Developers should design their applications to handle rate limit responses gracefully, possibly by implementing retry mechanisms with exponential backoff or queuing requests to process later.
Writing Your First Python Script to Integrate Open AI ChatGPT API
Integrating ChatGPT into a Python project begins with crafting a script that connects to the API and customizes its output. This process involves establishing a connection to the API and fine-tuning parameters to achieve desired responses.
Connecting to the Open AI ChatGPT API
To initiate a connection with the ChatGPT API, developers can utilize the openai
library in Python. Below is a straightforward example demonstrating how to set up this connection:
pythonCopy codeimport openai
# Replace 'your-api-key' with your actual OpenAI API key
openai.api_key = 'your-api-key'
response = openai.ChatCompletion.create(
model='gpt-4o-mini',
messages=[
{'role': 'system', 'content': 'You are a helpful assistant.'},
{'role': 'user', 'content': 'Hello, how can you assist me today?'}
]
)
# Extracting and printing the assistant's reply
assistant_reply = response['choices'][0]['message']['content']
print(assistant_reply)
In this script, the openai
library is imported, and the API key is set using openai.api_key
. The ChatCompletion.create
method is then called with the specified model and a list of messages that define the interaction. The response from the API is stored in the response
variable, from which the assistant’s reply is extracted and printed.
Customizing the Output
The ChatGPT API provides several parameters that allow developers to tailor the generated responses:
- Temperature: Controls the randomness of the output. Lower values (e.g., 0.2) make the output more focused and deterministic, while higher values (e.g., 0.8) introduce more diversity and creativity.
- Max Tokens: Sets the maximum length of the generated response in tokens. This helps in controlling the verbosity of the output.
By adjusting these parameters, developers can influence the behavior of the AI to better suit their application’s needs. For instance, setting a lower temperature can be beneficial for tasks requiring precise and consistent answers, whereas a higher temperature might be preferable for creative writing endeavors.
Experimenting with different prompt styles and parameter settings can yield varied results, enabling developers to fine-tune the AI’s responses to align with specific requirements.
Advanced Usage for Open AI ChatGPT API
Integrating ChatGPT into applications can be enhanced by creating a conversational bot and optimizing API usage. This involves maintaining context in interactions and implementing strategies for efficient processing.
Subsection 6.1: Creating a Conversational Bot
Developers can build a conversational bot using the ChatGPT API by preserving the context of interactions. This is achieved by maintaining a list of messages that includes the roles (‘user’, ‘assistant’, ‘system’) and their respective content. Here’s an example:
pythonCopy codeimport openai
openai.api_key = 'your-api-key'
# Initialize the conversation with a system message
messages = [
{'role': 'system', 'content': 'You are a helpful assistant.'}
]
while True:
user_input = input("User: ")
messages.append({'role': 'user', 'content': user_input})
response = openai.ChatCompletion.create(
model='gpt-4o-mini',
messages=messages
)
assistant_reply = response['choices'][0]['message']['content']
print(f"Assistant: {assistant_reply}")
messages.append({'role': 'assistant', 'content': assistant_reply})
In this script, the conversation starts with a system message that sets the assistant’s behavior. The messages
list is updated with each user input and the assistant’s reply, ensuring the context is preserved throughout the interaction.
Best Practices for Handling User Inputs and Responses
- Input Validation: Ensure user inputs are sanitized to prevent injection attacks or unintended behavior.
- Context Management: Limit the number of messages retained to manage token usage effectively, as the API has token limits that include both input and output tokens.
- User Feedback: Implement mechanisms to handle unclear inputs gracefully, possibly by asking clarifying questions.
Subsection 6.2: API Optimization Tips
Efficient use of the ChatGPT API involves strategies to reduce token usage and leverage batch processing.
Reducing Token Usage
- Prompt Engineering: Craft concise prompts to minimize token count. For example, instead of “Can you please provide information about the weather today?” use “Weather today?”
- Use of Stop Sequences and Token Limits: Implement stop sequences to signal the model when to stop generating text and set maximum token limits to prevent overly lengthy responses.
Leveraging the API Efficiently for Batch Processing
- Batch Requests: Combine multiple tasks into a single API call to maximize throughput. This approach is particularly useful when processing large datasets or performing bulk operations.
- Asynchronous Processing: Utilize asynchronous API calls to handle multiple requests concurrently, reducing overall processing time.
By implementing these practices, developers can create responsive conversational bots and optimize API usage, balancing performance with cost-effectiveness.
Troubleshooting Common Issues While Using Open AI ChatGPT API
Encountering errors while working with the OpenAI ChatGPT API in Python can be a common hurdle. Understanding these issues and their solutions can streamline the development process.
Authentication Failures often arise from incorrect or missing API keys. To resolve this, ensure that the API key is correctly set in the environment variables or directly within the code. If the key has expired or been revoked, generating a new one from the OpenAI dashboard is advisable. It’s also important to verify that the API key has the necessary permissions for the intended operations
Rate Limit Exceeded errors occur when the number of API requests surpasses the allowed threshold within a specific timeframe. To mitigate this, implement exponential backoff strategies, which involve retrying requests after progressively longer intervals. Monitoring the application’s request rates and optimizing code to reduce unnecessary calls can also help in staying within the rate limits.
Invalid Parameters errors result from malformed requests or missing required parameters. To address this, carefully review the API documentation to ensure all necessary parameters are included and correctly formatted. Validating input data before making requests can prevent such errors.
Best Practices for Using Open AI ChatGPT API
When working with OpenAI’s ChatGPT API, it’s essential to follow best practices to ensure both security and ethical compliance. This approach not only protects sensitive information but also aligns with OpenAI’s usage policies.
Safeguarding API Keys
API keys serve as unique identifiers that grant access to OpenAI’s services. To prevent unauthorized use, it’s crucial to handle these keys securely:
- Secure Storage: Store API keys in secure environments, such as environment variables or dedicated secret management tools, to prevent exposure.
- Avoid Client-Side Exposure: Refrain from embedding API keys directly into client-side code, like JavaScript or mobile applications, as this can lead to unintended exposure. Instead, route requests through a backend server where the API key remains protected.
- Regular Key Rotation: Periodically regenerate and update API keys to minimize the risk of unauthorized access. This practice ensures that even if a key is compromised, its validity is limited.
Avoiding Sensitive Data in Prompts
When interacting with the ChatGPT API, it’s advisable to avoid including sensitive information in prompts. While OpenAI implements measures to protect data, minimizing the sharing of confidential details reduces potential risks.
Ethical Considerations
Using the ChatGPT API responsibly involves adhering to ethical guidelines:
- Preventing Misuse: Ensure that applications do not generate harmful content, such as misinformation, hate speech, or material that violates privacy. Developers are encouraged to implement content moderation strategies to mitigate misuse.
- Compliance with OpenAI’s Usage Policies: Familiarize yourself with and adhere to OpenAI’s usage policies, which outline acceptable use cases and prohibited activities. This compliance is vital to maintain the integrity and safety of AI applications.
By integrating these best practices, developers can create applications that are secure, ethical, and aligned with OpenAI’s standards, fostering trust and reliability in AI-driven solutions.
Check out: Free LinkedIn Post Generator
Final Thoughts on How to Use Open AI ChatGPT API in Python
Incorporating OpenAI’s ChatGPT API into Python applications unlocks a world of possibilities, from crafting conversational agents to automating content creation.
This article offers a step-by-step guide on setting up the API, writing your first script, and exploring advanced features. Whether you’re a developer aiming to enhance your projects with AI capabilities or simply curious about the integration process, this guide provides the insights needed to effectively utilize the ChatGPT API in your Python endeavors.